Arduino - Bluetooth
In this tutorial, we are going to learn:
- How to use Bluetooth with Arduino
- How to send data from Arduino to a smartphone app via Bluetooth
- How to receive data on Arduino from the smartphone app via Bluetooth
- How to control Arduino from the smartphone app via Bluetooth
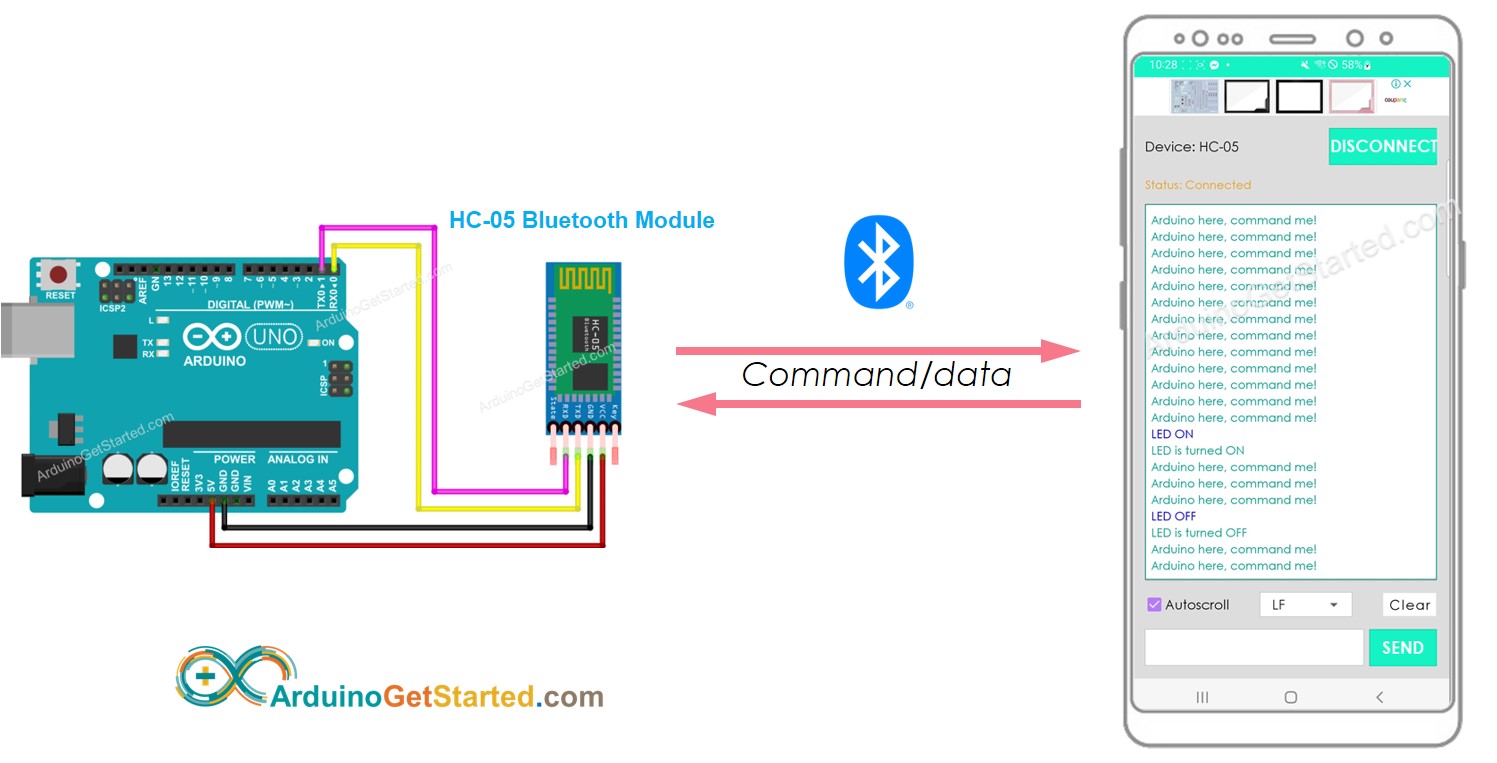
The scope of this tutorial:
- Arduino exchanges data with the smartphone app. The communication between two Arduino via Bluetooth will be presented in another tutorial.
- The HC-05 Bluetooth Module is used in this tutorial. This module is widely used and popular
- You can use any Android/iOS app. This tutorial will use Bluetooth Serial Monitor App on Android for demonstration.
- You can control anything connected to Arduino from the smartphone app. This tutorial will take LED and servo motor as examples.
This tutorial is about using Classic Bluetooth (Bluetooth 2.0). If you are looking for a Bluetooth Low Energy - BLE (Bluetooth 4.0), See this similar tutorial: Arduino - Bluetooth Low Energy
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
About HC-05 Bluetooth Module
HC-05 is a Serial Bluetooth module. It works as a Serial to Bluetooth Converter. It does:
- Receive data from the Serial RX pin and transmit the data to the paired device (such as a smartphone) via Bluetooth
- Receive data from Bluetooth (from the paired device) and send the data to the Serial TX pin.
More specifically, In the context of Arduino communicates with smartphone App (Android/iOS):
- Arduino connects to HC-05 Bluetooth Module via Serial/SoftwareSerial pins
- HC-05 Bluetooth Module is paired with a smartphone App
- Arduino sends the data to the smartphone App just by sending data to Serial/SoftwareSerial
- Arduino receives the data from the smartphone App just by reading data to Serial/SoftwareSerial
- No specific Bluetooth code is required on Arduino
Pinout
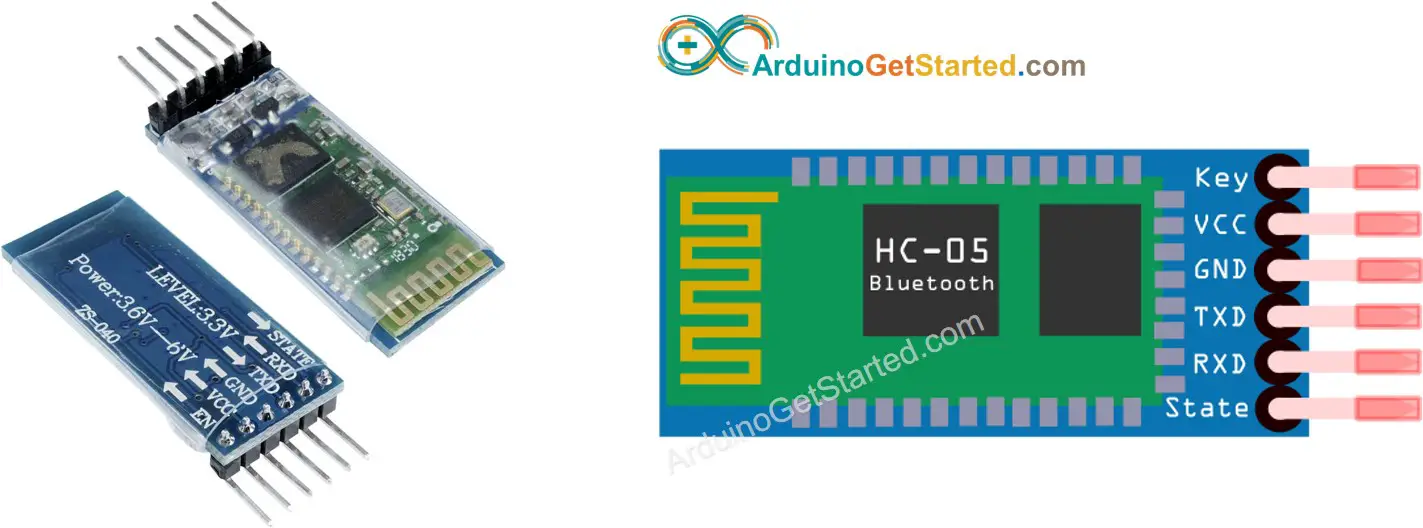
The HC-05 Bluetooth Module has 6 pins:
- Enable/Key pin: This pin is used to toggle between Data Mode (set LOW) and Command mode (set HIGH). If not connected, it is in Data mode by default
- VCC pin: power pin, connect this pin to +5V of Supply voltage
- GND pin: power pin, connect this pin to the GND of the power source
- TX pin: Serial data pin, connect this pin to the RX pin of Arduino. The data received via Bluetooth will be sent to this pin as serial data.
- RX pin: Serial data pin, connect this pin to the TX pin of Arduino. The data received from this pin will be sent to Bluetooth
- State: The state pin is connected to the onboard LED, it can be used as feedback to check if Bluetooth is working properly.
However, for basic functions, we just need to use 4 pins of The HC-05 Bluetooth Module to connect to Arduino.
The HC-05 Bluetooth Module also has two built-in components:
- A LED: indicates the status of Module
- Blinking once per 2 seconds: Module has entered Command Mode
- Blinking repeatedly: Waiting for connection in Data Mode
- Blinking twice per 1 second: Connection successful in Data Mode
- Button: can be used to control the Key/Enable pin to select the operation mode (Data or Command Mode)
How It Works
The HC-05 Bluetooth module has two operation modes:
- Data mode: is used to exchange data with the paired device
- Command Mode. is used to configure parameters.
Fortunately, The HC-05 Bluetooth module can work with Arduino by using the default setting without configuration.
HC-05 Default Settings
Default Bluetooth Name | “HC-05” |
---|---|
Default Password | 1234 or 0000 |
Default Communication | Slave |
Default Mode | Data Mode |
Default Data Mode Baud Rate | 9600, 8, N, 1 |
Default Command Mode Baud Rate | 38400, 8, N, 1 |
About Bluetooth Serial Monitor App
The Bluetooth Serial Monitor App is a mobile app that has the User Interface that looks like the Serial Monitor of Arduino IDE. It communicates with Arduino via Bluetooth. You can interact with Arduino via this app as if Serial Monitor on your PC, without adding any special code for the Bluetooth module in your Arduino code, by doing the following step:
- Connect Arduino to HC-05 Bluetooth module
- Install Bluetooth Serial Monitor App on your smartphone
- Open the App and pair it with the HC-05 Bluetooth module
And now you can send/receive data from Arduino just like the Serial Monitor of Arduino IDE. You do not need to modify the existing Arduino code or do not need to add any Bluetooth code to the new Arduino code.
Wiring Diagram
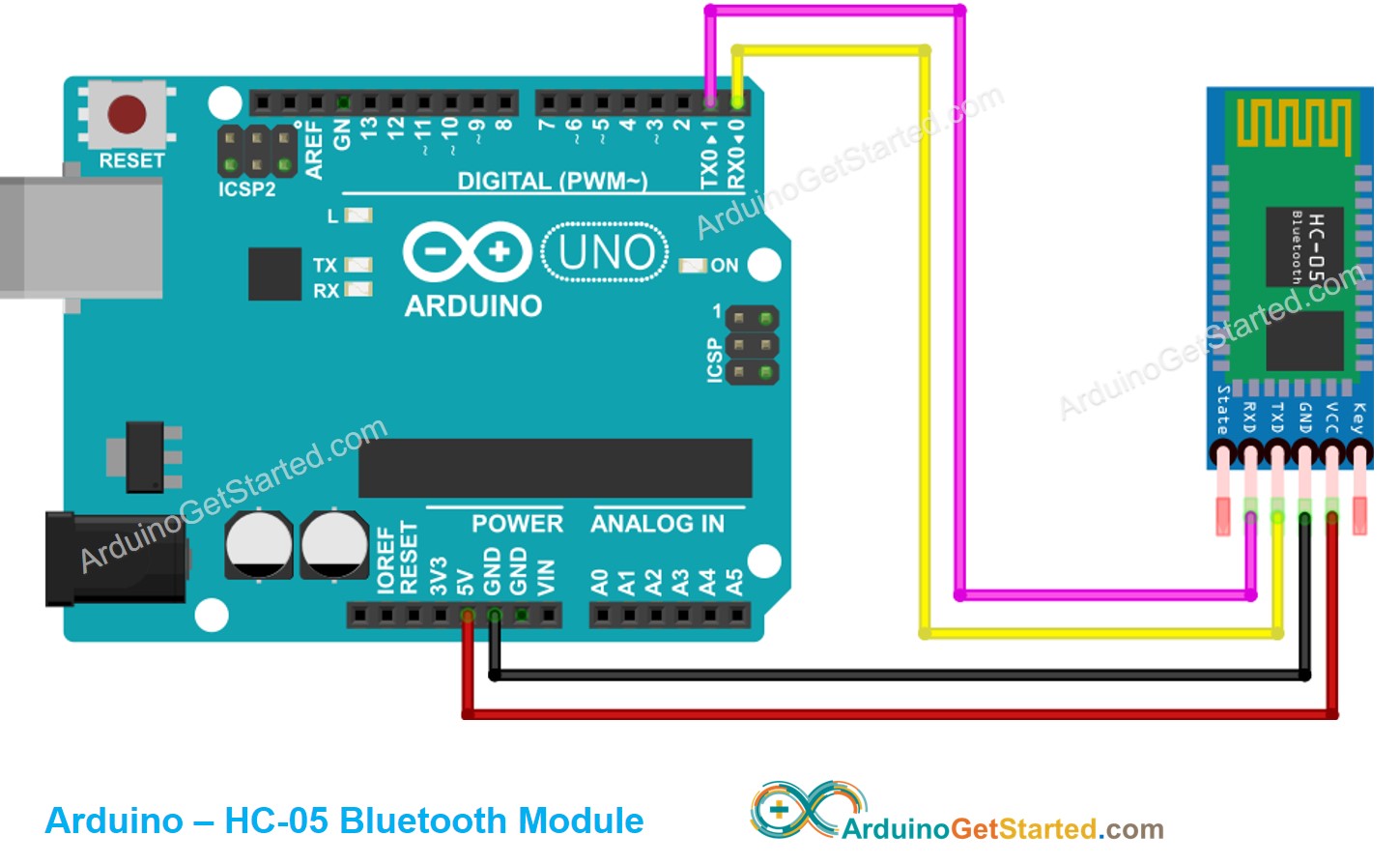
This image is created using Fritzing. Click to enlarge image
Wiring Table
Arduino Pins | HC-05 Bluetooth Pins |
---|---|
RX (Pin 0) | TX |
TX (Pin 1) | RX |
5V | VCC |
GND | GND |
Enable/Key (NOT connected) | |
State (NOT connected) |
※ NOTE THAT:
You can use other Arduino pins by changing the Serial object in the Arduino code to another Serial1, Serial2,..., or SoftwareSerial if available.
How To Program For Bluetooth
No Bluetooth-dedicated code is required. We just need to use the Serial code.
Arduino sends data to Bluetooth App on Smartphone
To send data from Arduino to Bluetooth App on Smartphone, we need to use the following Arduino code:
In this example, we will send the “Arduino here, command me!” from Arduino to Bluetooth App on Smartphone every second
Quick Steps
- Install Bluetooth Serial Monitor App on your smartphone
- Wire the HC-05 Bluetooth module to Arduino as above wiring diagram
- Copy the above code and open it with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino. You may be failed to upload the code to Arduino. If so, disconnect TX and RX pins from Bluetooth module, upload the code, and then reconnect RX/TX pin again.
- Open Serial Monitor on Arduino IDE
- Open Bluetooth Serial Monitor App on your smartphone
- Select the Classic Bluetooth mode
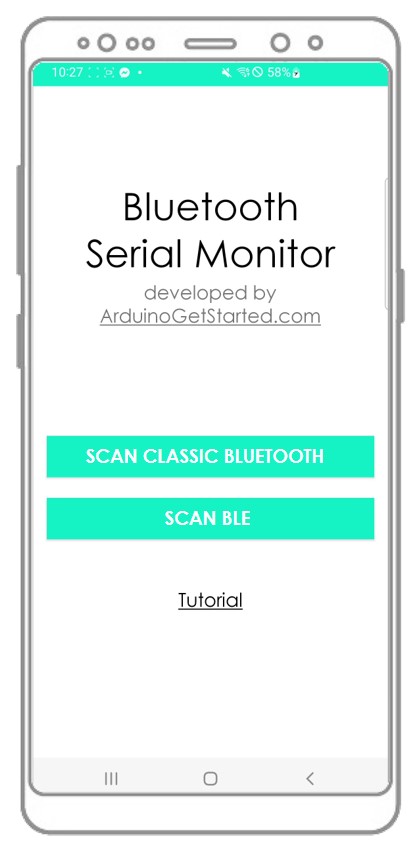
- Pair it with HC-05 Bluetooth module
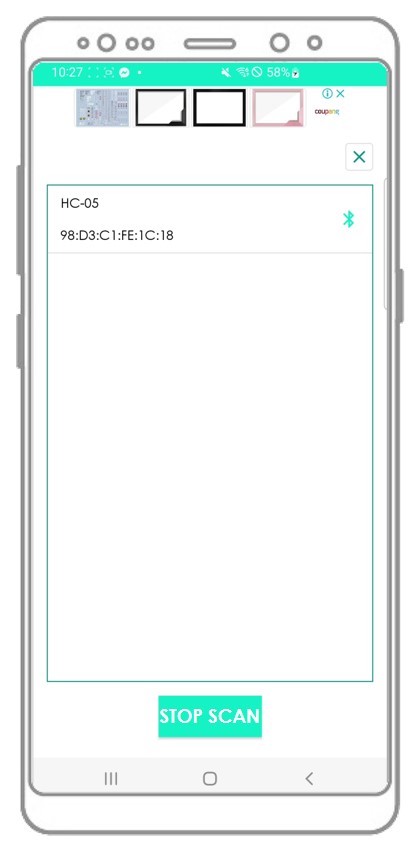
- See the result on the Android App.
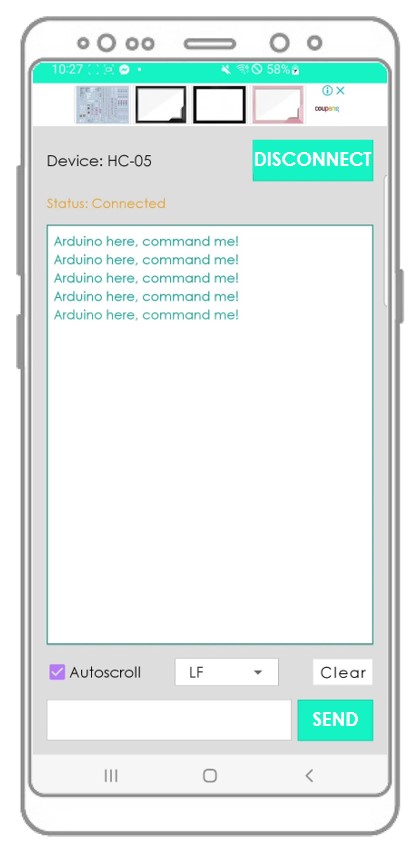
- See the result on the Serial Monitor of Arduino IDE.
You will see that the data on the Serial Monitor of Arduino IDE and on the Android App are identical
Bluetooth App Send data To Arduino
The below code does:
- Bluetooth App Send data To Arduino
- Arduino reads data and sends the response back to Bluetooth:
Quick Steps
- Copy the above code and open it with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Open Serial Monitor on Arduino IDE
- Open Android App and pair it with the HC-05 Bluetooth module (similar to the previous example)
- After connecting, Type "LED ON" or "LED OFF" on the Android App and click the "SEND" button
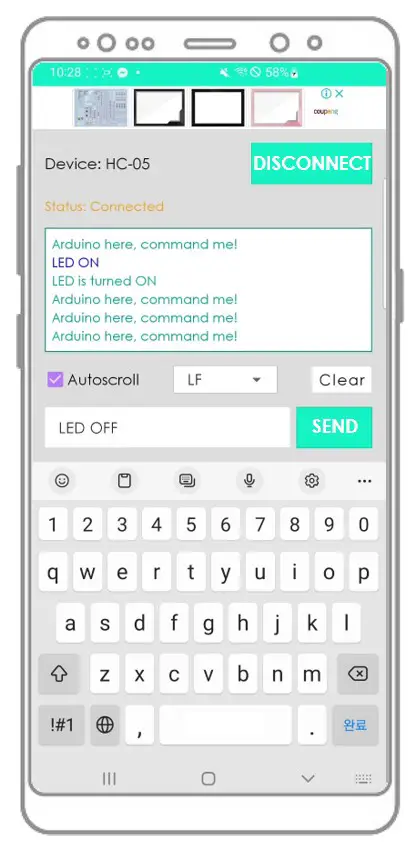
- Arduino receives the data and prints the response to the Serial port. This data will be sent to the Bluetooth app
- See the result on the Android App
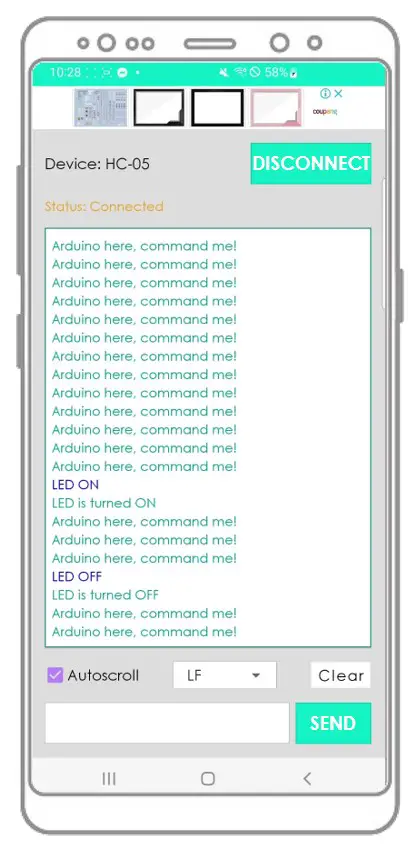
- See the result on the Serial Monitor of Arduino IDE
You will see that the data on the Serial Monitor of Arduino IDE and on the Android App are identical
Arduino Code - Control LED with smartphone App via Bluetooth
The below Arduino example code accepts two commands (“ON” and “OFF”) from Bluetooth Serial Monitor App to turn on/off a built-in LED.
You can see the instructions in more detail in Arduino controls LED via Bluetooth/BLE tutorial
Arduino Code - Control Servo Motor with smartphone App via Bluetooth
The below Arduino code received the angle value from Bluetooth Serial Monitor App to control the angle of the servo motor.
You can see the instructions in more detail in Arduino controls Servo Motor via Bluetooth/BLE tutorial
If the Bluetooth Serial Monitor app is useful for you, please give it a 5-star rating on Play Store. Thank you!
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.